Intro
Font Awesome provides website icons used by hundreds of thousands of projects. If you have used the icons in the past or found an icon that would fit well in your project, you might find yourself wanting to use them in a Streamlit web app.
Until now there hasn’t been a guide for making Font Awesome and Streamlit work together.
Font Awesome’s docs assume you’re working in an environment with <script>
tags readily available, which isn’t the case in Streamlit.
While you can add HTML and CSS to the Markdown used by Streamlit, scripts are not run.
Despite these challenges there are still two methods to use Font Awesome icons in your streamlit app: a preferred method using CSS, and a backup method using Streamlit Components. Example code showing how you can use each method (and for generating the images in this post) can be found here.
Method 1: Import Icons with CSS
The first way to get Font Awesome icons into your app is to avoid using script tags altogether by importing Font Awesome’s CSS from an existing library. In the example below, we use a version stored in the cdnjs.
import streamlit as st
css_example = '''
I'm importing the font-awesome icons as a stylesheet!
<link rel="stylesheet" href="https://cdnjs.cloudflare.com/ajax/libs/font-awesome/6.0.0/css/all.min.css">
<i class="fa-solid fa-square"></i>
<i class="fa-solid fa-dragon"></i>
<i class="fa-solid fa-paw"></i>
'''
st.write(css_example, unsafe_allow_html=True)
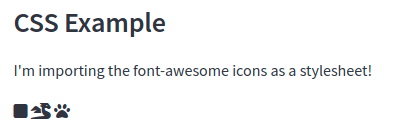
While this method works well, it’s not the safest.
As always, when using code from an external source, we’re trusting the host and the author not to upload anything malicious.
Additionally, since we’re adding HTML tags to our Markdown, we have to use the unsafe_allow_html
parameter in Streamlit.
Such custom HTML can potentially leave us open to HTML injection attacks (see this discussion for more details).
For my uses, the risks posed by pulling Font Awesome from a website are acceptable, but your own appetite for risk may vary.
Method 2: Using a Streamlit HTML Component
If you want a more secure method for using Font Awesome icons (or HTML in general), a Streamlit HTML Component is probably the right choice for you. Using HTML Components puts any scripts you want to run in an IFrame to isolate them from the rest of your Streamlit app. This method gives you much more freedom to include whatever you want in your app, but not without drawbacks.
Because your Component is isolated from the rest of the app, it doesn’t inherit its CSS. As a result, you’ll have to put in some work (and CSS) to make whatever lives in your Component have the same style as everything else. The other main drawback of an HTML Component is that it’s in HTML. While the rest of your app’s text is written and styled in Markdown, whatever lives in the Component will have to be written in HTML.
There are good reasons to use an HTML Component, though. For example, if you want to use paid icons you’ll have to go the Component route. Alternatively, if you have a Font Awesome kit you want to use, you’ll need to use script tags and, by extension, HTML Components.
If you decide to go the Component route, here’s example code to get you started:
<!-- awesome.html -->
<script src="https://kit.fontawesome.com/2c74303849.js" crossorigin="anonymous"></script>
<p> I'm in an HTML component containing fontawesome icons from a kit! I don't inherit CSS automatically!</p>
<p>
<i class="fa-solid fa-square"></i>
<i class="fa-solid fa-dragon"></i>
<i class="fa-solid fa-paw"></i>
</p>
import streamlit as st
def load_text(file_path):
"""A convenience function for reading in the files used for the site's text"""
with open(file_path) as in_file:
return in_file.read()
if __name__ == '__main__':
# Other demo code omitted for clarity
st.write('### Component Example')
# HTML component
component_example = load_text('awesome.html')
st.components.v1.html(component_example)
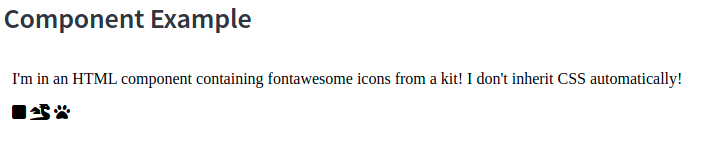
Conclusion
Hopefully you will now be able to use Font Awesome icons on your Streamlit app to make your fonts more awesome and your app more lit. Let me know if you know of workarounds for styling in HTML Components, or if you have another method you prefer to use to get icons in your app.